Available with: All-In-One or by request for other packages.
Consumption: Each request consumes 10 API calls regardless of history length.
The US Stock Market Ticks Data API provides granular tick data for all US equities spanning across all exchanges.
It includes current and delisted companies, encompassing approximately 80,000 tickers, 16,000+ securities are updated daily. The total data size is approximately 10 terabytes.
The dataset begins from January 1st, 2008, and is updated until the current date.
Quick Start
To get historical stock tick data, use the following URL:
https://eodhd.com/api/ticks/?s=AAPL&from=1694354400&to=1694455200&limit=1&api_token=demo&fmt=json
curl --location "https://eodhd.com/api/ticks/?s=AAPL&from=1694354400&to=1694455200&limit=1&api_token=demo&fmt=json"
$curl = curl_init();
curl_setopt_array($curl, array(
CURLOPT_URL => 'https://eodhd.com/api/ticks/?s=AAPL&from=1694354400&to=1694455200&limit=1&api_token=demo&fmt=json',
CURLOPT_RETURNTRANSFER => true,
CURLOPT_ENCODING => '',
CURLOPT_MAXREDIRS => 10,
CURLOPT_TIMEOUT => 0,
CURLOPT_FOLLOWLOCATION => true,
CURLOPT_HTTP_VERSION => CURL_HTTP_VERSION_1_1,
CURLOPT_CUSTOMREQUEST => 'GET',
));
$data = curl_exec($curl);
curl_close($curl);
try {
$data = json_decode($data, true, 512, JSON_THROW_ON_ERROR);
var_dump($data);
} catch (Exception $e) {
echo 'Error. '.$e->getMessage();
}
import requests
url = f'https://eodhd.com/api/ticks/?s=AAPL&from=1694354400&to=1694455200&limit=1&api_token=demo&fmt=json'
data = requests.get(url).json()
print(data)
library(httr)
library(jsonlite)
url <- 'https://eodhd.com/api/ticks/?s=AAPL&from=1694354400&to=1694455200&limit=1&api_token=demo&fmt=json'
response <- GET(url)
if (http_type(response) == "application/json") {
content <- content(response, "text", encoding = "UTF-8")
cat(content)
} else {
cat("Error while receiving data\n")
}
- s – symbol, for example, AAPL.US, consists of two parts: {SYMBOL_NAME}.{EXCHANGE_ID}. This API works only for US exchanges for the moment, then you can use ‘AAPL’ or ‘AAPL.US’ to get the data as well for other US tickers.
- api_token – your own API KEY, which you will get after you subscribe to our services.
- from and to – use these parameters to filter data by datetime. Parameters should be passed in UNIX time with UTC timezone, for example, these values are correct: “from=1694354400&to=1694455200” and correspond to ‘ 2023-09-10 14:00:00 ‘ and ‘ 2023-09-11 18:00:00 ‘.
- limit – the maximum number of ticks to be provided.
For testing purposes, you can try the following API Key (works only for AAPL.US ticker): demo
Output
We do provide output in JSON:
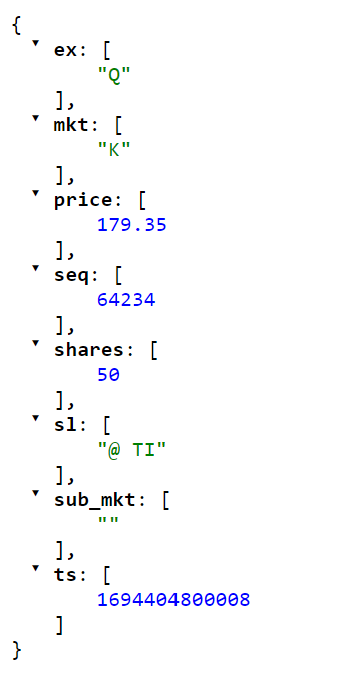
Where the fields are:
- ex – the exchange ticker is listed on
- mkt – market where trade took place
- price – the price of the transaction
- seq – trade sequence number
- shares – shares in transaction
- sl – sales condition
- ts -timestamp
- sub_mkt – sub-market where trade took place
Exchange codes
‘X’: ‘NASDAQ’,
‘K’: ‘CBOE’,
‘S’: ‘OTC’,
‘u’: ‘OTC’,
‘U’: ‘OTC’,
‘?’: ‘OTC’,
‘a’: ‘OTC’,
‘V’: ‘IEX’,
‘Y’: ‘CBOE’,
‘T’: ‘NASDAQ’,
‘J’: ‘CBOE’,
‘N’: ‘NYSE’,
‘C’: ‘NYSE’,
‘P’: ‘NYSE’,
‘A’: ‘NYSE’,
‘B’: ‘NASDAQ’,
‘W’: ‘CBOE’,
‘Q’: ‘NASDAQ’,
‘Z’: ‘CBOE’,
‘R’: ‘NASDAQ’
Trading codes
@ – Regular Sale
A – Acquisition
B – Bunched Trade
C – Cash Sale
D – Distribution
E – Placeholder
F – Intermarket Sweep
G – Bunched Sold Trade
H – Price Variation Trade
I – Odd Lot Trade
K – Rule 155 Trade (AMEX)
L – Sold Last
M – Market Center Official Close
N – Next Day
O – Opening Prints
P – Prior Reference Price
Q – Market Center Official Open
R – Seller
S – Split Trade
T – Form T
U – Extended trading hours (Sold Out of Sequence)
V – Contingent Trade
W – Average Price Trade
X – Cross/Periodic Auction Trade
Y – Yellow Flag Regular Trade
Z – Sold (out of sequence)
You can also check our Intraday Data API and Real-Time Data API via WebSockets.